- BLOG / NEWS
Building an Interactive Flight Analysis Dashboard with Dash.jl
By Maja Gwozdz | May 14, 2024
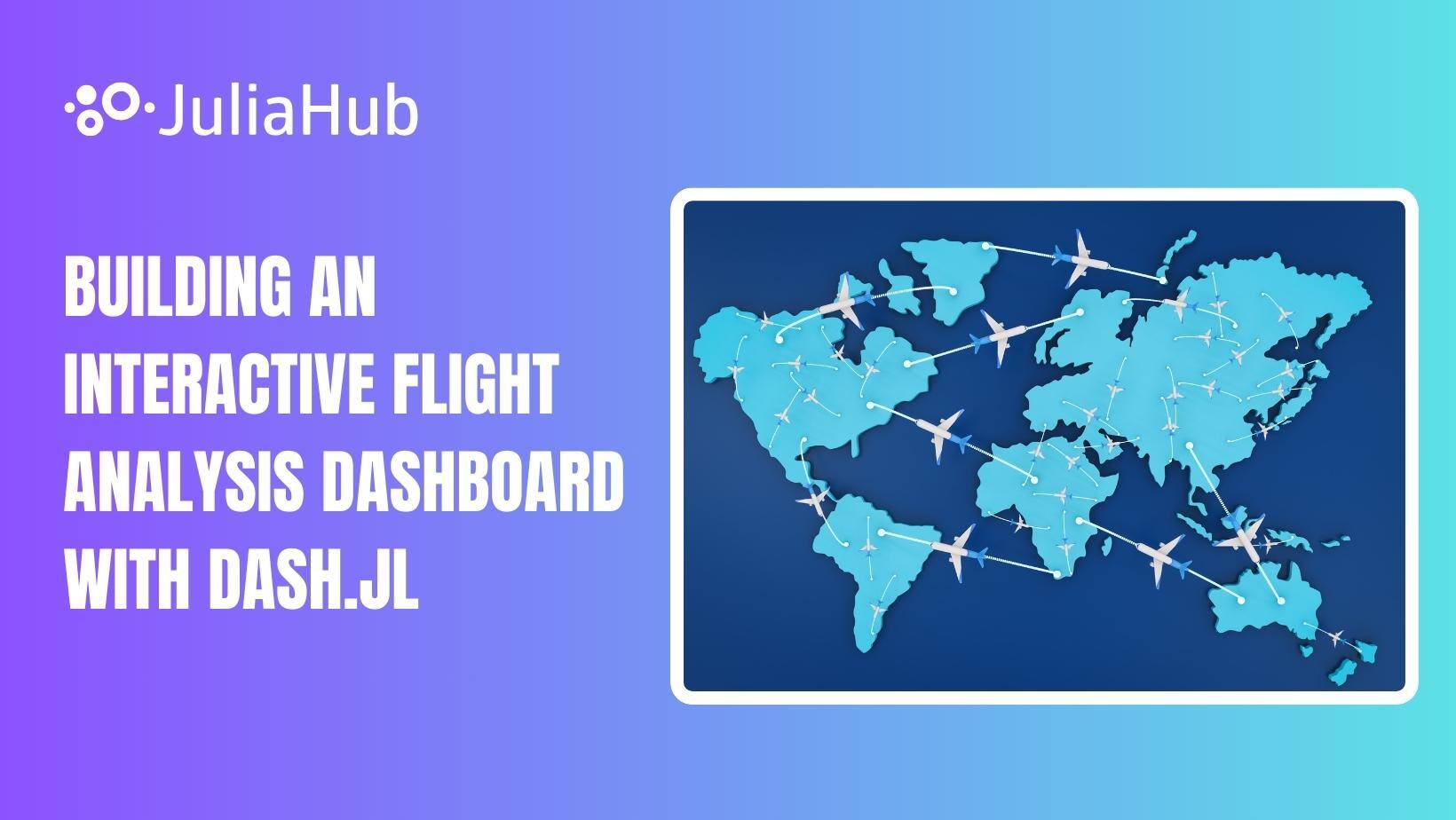
Welcome to our exploration of building an interactive web dashboard using Dash.jl, a Julia framework that allows for the creation of highly interactive, web-based data visualizations. This post will guide you through the development of a simple flight analysis dashboard. We will focus on combining interactive components and experimenting with different map projections. You can easily adapt this code to your own project. Let's get started!
Dashboard Details
The flight analysis dashboard is designed to provide the visualization of flight data. Users can easily interact with the data through various controls like dropdown menus for aircraft type selection, radio buttons for choosing map projections, and dynamic maps that display flight paths and details based on user inputs.
You can download the dataset and the final code.
Our Dash.jl application will look like this:
The dataset is very basic and contains fictional flight details. Here is an excerpt:
Key Features
-
Dynamic Filtering: Users can select specific aircraft types to visualize only the flights corresponding to those types.
-
Interactive Maps: The dashboard uses PlotlyJS to render geographical data interactively, allowing users to explore different views and details of the flights.
-
Responsive Design: Built with the responsive capabilities of Dash.jl, the dashboard adjusts to different user inputs in real-time, providing an engaging user experience.
By the end of this blog, you will have a comprehensive understanding of how to leverage Julia and Dash.jl to build an interactive web application for flight data analysis. Let's dive into the details and start building our dashboard!
Setting Up the Layout
In this section, we'll dive into setting up the layout for a flight analysis dashboard using Dash.jl, focusing on how the layout and interactive components are constructed to make data exploration easier.
First, let's include the relevant packages in the first line of our code:
using Dash, DashHtmlComponents, DashCoreComponents, CSV, DataFrames, PlotlyJS
Converting the dataset
Our dataset is in the CSV format. Let's turn it into a dataframe to make data manipulation easier:
# Load the dataset
df = CSV.File("path_to_dataset.csv") |> DataFrame
Once we've specified the data, let's add this line to our code:
app = dash()
This line initializes a new Dash application.
Configuring the Application Layout
The layout of the Dash application is where the components that make up the user interface are defined. If you have previous experience with HTML, you'll find component configuration really intuitive.
app.layout = html_div() do
[
html_h1("Flight Analysis"),
html_div("Dash.jl webinar"),
dcc_dropdown(
id="aircraft-type-dropdown",
options=[
Dict("label" => "All", "value" => "All");
[Dict("label" => aircraft_type, "value" => aircraft_type) for aircraft_type in unique(df[!, :AircraftType])]
],
value="All" # Default value
),
dcc_radioitems(
id="projection-type-selector",
options=[
Dict("label" => "Equirectangular", "value" => "equirectangular"),
Dict("label" => "Orthographic", "value" => "orthographic"),
],
value="equirectangular" # Default value
),
dcc_graph(id="flight-map"),
html_div(id="flight-details")
]
end
The components
We will focus on the interactive components in the next section. At this point, let's look at the basic building blocks:
-
Header and Subheader:
-
html_h1("Flight Analysis")
: Creates a header (h1 HTML tag) for the title of the dashboard. -
html_div("Dash.jl webinar")
: Adds a division (div HTML tag) with text, serving as a subheader or additional information.
-
-
Graph and Details Section:
-
dcc_graph(id="flight-map")
: Defines a space for Plotly graphs; in this case, a map that will display flights based on the selected filters. -
html_div(id="flight-details")
: An empty division that can be used to display additional details about specific flights when a user interacts with the map.
-
-
dcc components:
-
By integrating these interactive components, users can adjust the flight map display according to their needs. In our example, the dropdown allows for specific data filtering, while the radio buttons enable switching between different visual representations of the geographical data.
-
Dropdown for Aircraft Type Selection
dcc_dropdown(
id="aircraft-type-dropdown",
options=[
Dict("label" => "All", "value" => "All");
[Dict("label" => aircraft_type, "value" => aircraft_type) for aircraft_type in unique(df[!, :AircraftType])]
],
value="All" # Default value
)
Code explanation
-
ID: Identifies this dropdown uniquely within the app, allowing it to be used by callbacks.
-
Options: Populated dynamically using a list comprehension that iterates through each unique aircraft type obtained from the dataset. This list is prefixed with an option labeled "All", which allows users to select all aircraft types.
-
Dict("label" => "All", "value" => "All")
: Adds an option for displaying all data. -
Comprehension: Generates a dictionary for each aircraft type where the
label
is the human-readable text shown in the dropdown, andvalue
is the corresponding value used in the data.
-
-
Value: Sets "All" as the default selection so that initially, the dashboard displays data for all aircraft types.
Radio Items for Map Projection Selection
dcc_radioitems(
id="projection-type-selector",
options=[
Dict("label" => "Equirectangular", "value" => "equirectangular"),
Dict("label" => "Orthographic", "value" => "orthographic"),
],
value="equirectangular" # Default value
)
Code explanation
-
ID: Again, as with the dropdown above, it uniquely identifies the group of radio items within the dashboard.
-
Options: This array contains two dictionaries, each representing a different type of map projection available for displaying the geographical data.
-
Equirectangular
: This projection represents a standard map projection used commonly in world maps. -
Orthographic
: Provides a globe-like, spherical view of the Earth, which can be useful for a different visual perspective.
-
-
Value: The default projection is set to "equirectangular", so this view is loaded when the dashboard is initially displayed.
Understanding Callbacks
In this part, we explore how callbacks are used in our Dash.jl application to dynamically update a flight map based on user inputs. This functionality is central to interactive data visualization, and leads to a responsive interface that adapts to changes in user preferences.
Setting Up the Callback
callback!(app, Output("flight-map", "figure"),
[Input("aircraft-type-dropdown", "value"), Input("projection-type-selector", "value")])
As a general tip, try to use meaningful names for inputs, outputs, and figures. Even if your Dash application is concise, this convention will help you avoid simple bugs.
Components of the Callback
-
Target Output:
Output("flight-map", "figure")
specifies that the output of the callback will be the figure property of the component with the ID "flight-map". This component is a graph that will display the flights map. -
Inputs: The callback listens to changes in two inputs:
-
Input("aircraft-type-dropdown", "value")
captures changes in the selected aircraft type from the dropdown. -
Input("projection-type-selector", "value")
tracks changes in the selected map projection from the radio items.
-
The Callback Function
Inside the callback function, the inputs are used to filter and display the data accordingly.
do aircraft_type, selected_projection
filtered_df = if aircraft_type == "All"
df
else
filter(row -> row.AircraftType == aircraft_type, df)
end
Data Filtering
-
All Aircraft Types: If "All" is selected, the entire dataset is used.
-
Specific Aircraft Type: If a specific type is chosen, the dataset is filtered to only include flights of that type. This is done using the
filter
function with a conditional that checks theAircraftType
of each row against the selected type.
Creating the Map Visualization
Let's adjust our map parameters:
map_trace = scattergeo(lat=filtered_df[!, "Latitude"], lon=filtered_df[!, "Longitude"], mode="markers",
text=filtered_df[!, "FlightID"], marker=Dict(:size=>10, :color=>"red"))
layout = Layout(title="Flights Map", geo=Dict(:scope=>"world", :showland=>true, :landcolor=>"rgb(243, 243, 243)",
:countrycolor=>"rgb(204, 204, 204)", :showcountries=>true,
:projection=>Dict(:type=>selected_projection)))
-
Map Trace: Defines how the data points (flights) are plotted on the map. Each point is marked by its geographical coordinates with additional styling (red color, size of markers).
-
Layout: Sets up the overall appearance of the map, including map projection, colors, and whether to show country borders and land.
Return the Plot
return PlotlyJS.plot([map_trace], layout)
Almost finished! This line generates the updated map based on the filtered data and layout specifications, and returns it as the new figure for the "flight-map" graph component.
To run the application, navigate to the Julia REPL and type:
include("path_to_your_dash_app.jl")
We hope this post inspires you to consider Julia and Dash.jl for your next data visualization project, unlocking new possibilities in data exploration and interactive web development!
Looking for Model Predictive Control (MPC)?
Learn about JuliaSim Control in our webinar on MPC, trimming, and linearization in JuliaSim.
Watch NowRECENT POSTS
-
JuliaCon 2024 Recap
Andrew Claster • Jul 24, 2024
-
JuliaSim Studio: Unmitigated Control for the Power Modeler
Chris Rackauckas • Jul 19, 2024
-
Quick and Easy Data Migration with JuliaHub’s New Tool
Deep Datta • Jul 19, 2024